Everyone’s reporting needs are different, and though Microsoft provides many great reports out of the box, they can’t fit the needs of every business at once. Therefore, most businesses will want to customize at least some of their reports. Luckily, Microsoft has made the process of customizing their reports fairly straightforward – you just make a copy of the existing report and change the design as needed, extending any data provider classes you need to along the way. The question, though, is how you replace the standard report with your new masterpiece?
The answer to this question isn’t quite as straightforward. Looking in print management, you’ll see a dropdown for Report Format with the standard report listed, but not your customized version. So how does this this dropdown get populated?
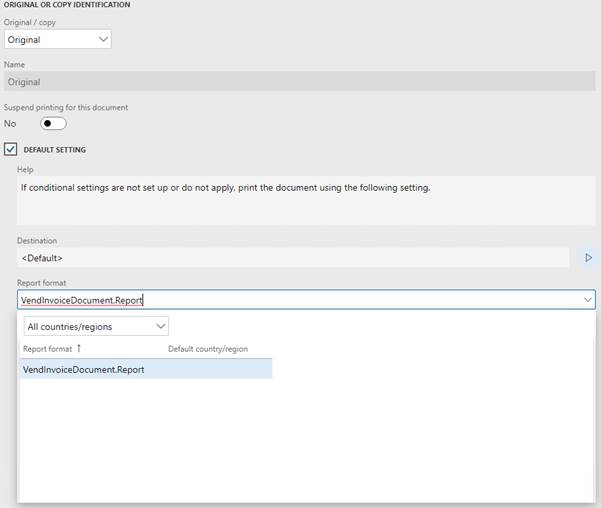
To get your report to show up in this dropdown, you will need to extend the PrintMgmtDelegatesHandler class.
In this example, I have customized the vendor invoice to remove the logo and replace it with the word Demo. When I go to the Invoice Journal and run the report through Preview/Print -> Original preview, I get the following report.
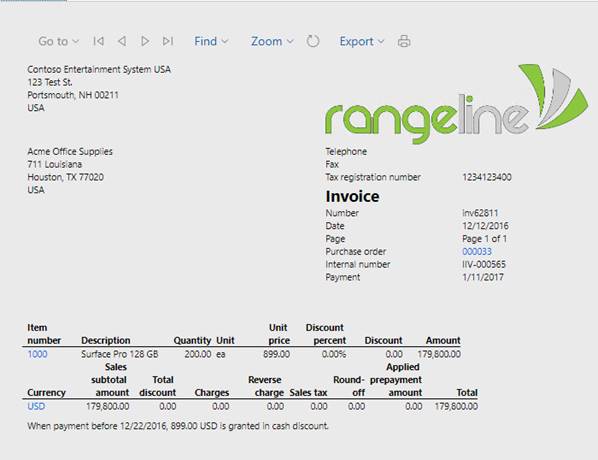
Now I want to extend the PrintMgmtDelegatesHandler class with the following code. This adds a chain of command method for the getDefaultReportFormatDelegateHandler method that runs after the standard method so we can overwrite the standard report assignment.
/// <summary>
/// Extension of <c>PrintMgmtDelegatesHandler</c> class.
/// </summary>
[ExtensionOf(classStr(PrintMgmtDelegatesHandler))]
final class RangelinePrintMgmtDelegatesHandler_Extension
{
/// <summary>
/// Extension for the getDefaultReportFormatDelegateHandler method of the <c>PrintMgmtDelegatesHandler</c> class.
/// </summary>
/// <param name = "_docType"><c>PrintMgmtDocumentType</c> enumeration value.</param>
/// <param name = "_result">The <c>EventHandlerResult</c> object.</param>
public static void getDefaultReportFormatDelegateHandler(PrintMgmtDocumentType _docType, EventHandlerResult _result)
{
PrintMgmtReportFormatName formatName;
next getDefaultReportFormatDelegateHandler(_docType, _result);
formatName = PrintMgmtDelegatesHandler::rangelineGetDefaultReportFormat(_docType, _result.result());
if (formatName)
{
_result.result(formatName);
}
}
/// <summary>
/// Gets the report format value.
/// </summary>
/// <param name = "_docType">The <c>PrintMgmtDocumentType</c> enumeration value.</param>
/// <returns>The report format value.</returns>
private static PrintMgmtReportFormatName rangelineGetDefaultReportFormat(
PrintMgmtDocumentType _docType,
PrintMgmtReportFormatName _origFormatName)
{
PrintMgmtReportFormatName formatName;
switch (_docType)
{
case PrintMgmtDocumentType::PurchaseOrderInvoice:
if (_origFormatName == ssrsReportStr(VendInvoiceDocument, Report))
{
formatName = ssrsReportStr(RangelineVendInvoiceDocument, Report);
}
break;
}
return formatName;
}
}
If I go back to print management now, I see my new report added to the dropdown list.
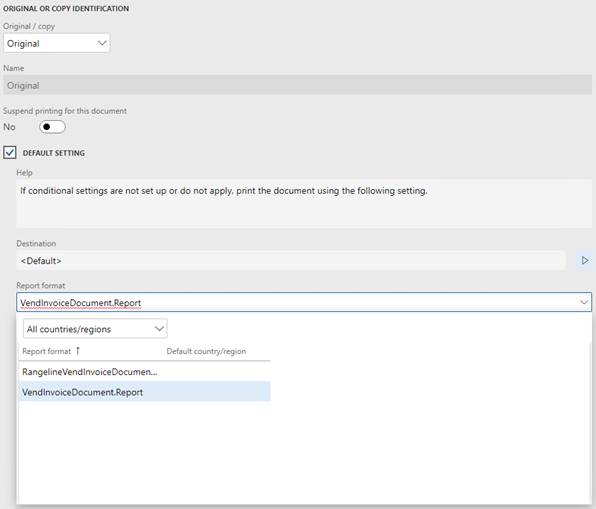
And when I run the report from Preview/Print -> Original preview, I get my newly customized report.
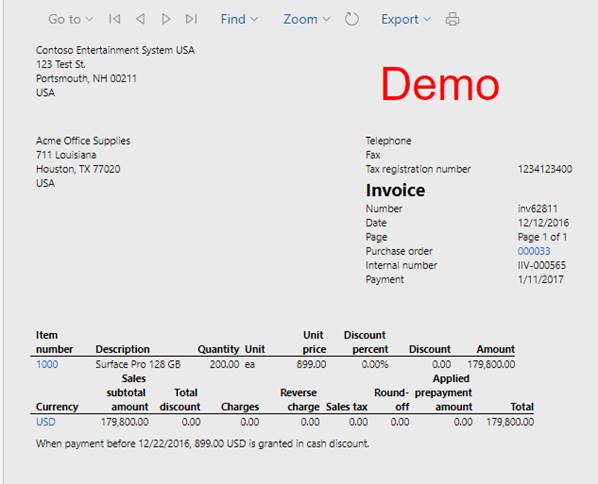